I want to validate the data received from branch offices. They provide totalstockreceivedother,truckstocks,railstocks. I want to validate whether truck stocks and rail stocks add up to the value equal to totalstockreceivedother.So I created another value totalstockreceived and try to print the boolean value.
My Code is as follows
totalstockreceivedother=1000
truckstocks=600
railstocks=400
totalstockreceived=truckstocks+railstocks
if(totalstockreceivedother=totalstockreceived,true,false)
print(boolean(if(totalstockreceivedother==totalstockreceived,true,false)))
It is returning error:SyntaxError: invalid syntax. Maybe you meant ‘==’ or ‘:=’ instead of ‘=’?
Help me.
Hi @Jagadeesan_Ravichand ,
totalstockreceivedother = 1000
truckstocks = 600
railstocks = 400
totalstockreceived = truckstocks + railstocks
if totalstockreceivedother == totalstockreceived:
print(True)
else:
print(False)
To check the equality, you should use == (double equalto symbol). Single = symbol will work as assignment.
மேலும் bool மற்றும் Str function மூலமாகவும் bool value ஐ print செய்யலாம் என்றும் அறிந்து கொண்டேன்.
1 Like
Great!
Also remember, print will implicitly call str to convert everything to string. So,
totalstockreceivedother = 1000
truckstocks = 600
railstocks = 400
totalstockreceived = truckstocks + railstocks
print(totalstockreceivedother == totalstockreceived)
this will also produce same results.
The easier method is:
totalstockreceivedother = 1000
truckstocks = 600
railstocks = 400
totalstockreceived = truckstocks + railstocks
print(totalstockreceivedother == totalstockreceived)
bool operator இல்லாமலேயே print function,bool value ஐ அச்சிடும் நிலையில், வேறு எந்த சூழ்நிலையில் bool பயன்பாடு தேவைப்படும் என விளக்க முடியுமா
Good Question,
In situations, where your function returns a non boolean value. Like numbers, strings, list. In those situation if you need to get a boolean value, then you can perform bool operation.
Numbers: 0 → False; Other than 0 → true.
print(bool(12))
print(bool(0))
Strings: “” → False, Non-Empty Strings → True
print(bool("")) # False
print(bool("parottaSalna")) # True
Similarly to all the data structures, if the list, tuple or dict is empty then it will return false.
Another example,
def check_value():
value = bool(value)
if value:
print("Value is True")
else:
print("Value is False")
check_value(None) # Value is False
check_value(10) # Value is True
check_value(None)
ல் None என்பது Non-empty string தானே. பிறகு எப்படி False என output வரும்?
None is not a string. Its a None type.
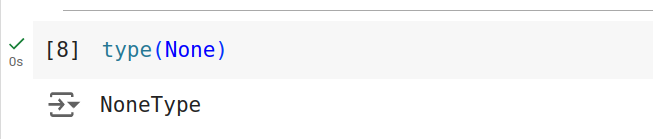
So it will result in False.
None is False.